<9:30 1교시>
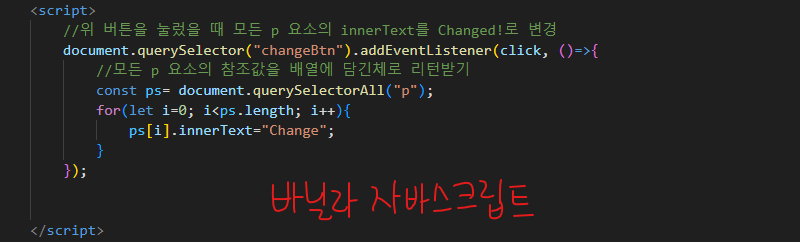
어떤 라이브러리도 이용하지 않고 코딩한 것 : 바닐라 자바스크립트
jquery : 라이브러리를 이용해서 코딩된 자바스크립트
jsp에서 jquery를 사용하는 게 괜찮다(단, 바닐라 자바스크립트를 할줄 안다는 가정 하에)
jquery 여러 웹 브라우저가 상호호환이 잘 안되던 시절이 있는데, 이때 많이 사용했고,
우리가 유지보수할 때 +jsp 쓸 때 사용할 수 있기 때문에 배운다.
https://jquery.com/
jQuery
What is jQuery? jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers.
jquery.com
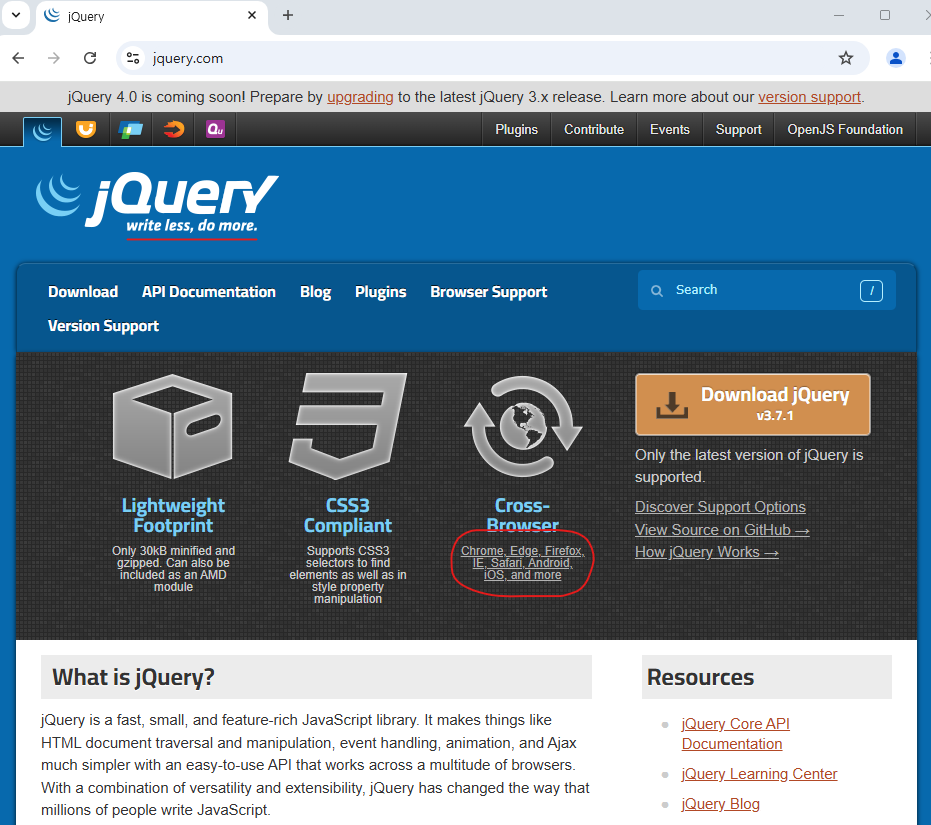
Download>CDN > jquerydelivr.cdn 카피해서 스크립트에 복붙

$(요소명선택자).action( ).action2( ).....
jquery를 로딩하면 $라는 이름으로 jqeury의 기능을 모두 가진 특별한 배열을 반환하는 함수가 생기는데, 이 $ 함수를 호출하고, 문서 객체의 요소명선택자를 다 쓸 수 있고, 여러 개의 동작을 .동작.동작.동작 식으로 연달아 실행할 수 있음.
위에서 p 요소의 innerText를 모두 변경하는 동작을 하기 위한 jquery 코딩은
$("#changeBtn2").on("click", ()=>{
$("p").text("clicked");
});
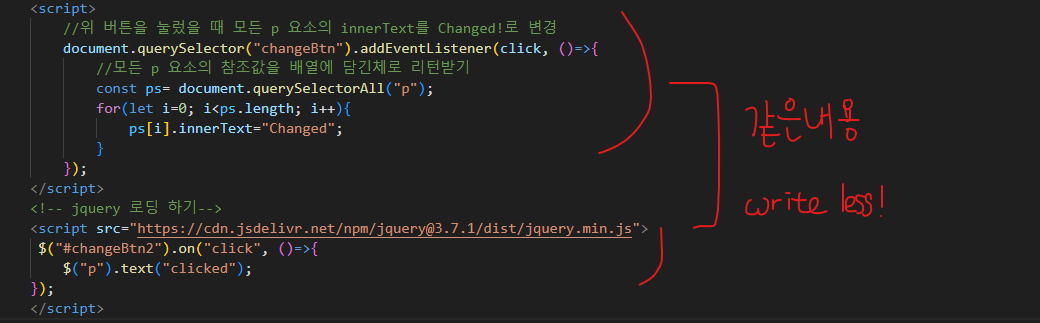
이렇게 짧게 끝낼 수가 있다.(다시 말하지만 이럴거면 코딩을 왜 배우는거야)
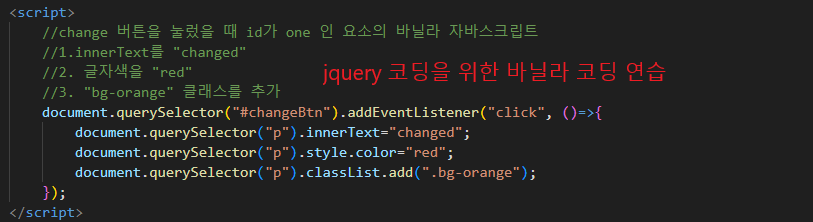

<10:30 2교시>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
.bg-orange{
background-color: orange;
}
</style>
</head>
<body>
<p>p1</p>
<p class="my-class">p2</p>
<p class="my-class">p3</p>
<p class="my-class">p4</p>
<p id="one">p5</p>
<button id="changeBtn">change</button>
<button id="changeBtn2">change2</button>
<script src="https://cdn.jsdelivr.net/npm/jquery@3.7.1/dist/jquery.min.js"></script>
<script>
//change 버튼을 눌렀을 때 id가 one 인 요소의 바닐라 자바스크립트
//1.innerText를 "changed"
//2. 글자색을 "red"
//3. "bg-orange" 클래스를 추가
document.querySelector("#changeBtn").addEventListener("click", ()=>{
document.querySelector("p").innerText="changed";
document.querySelector("p").style.color="red";
document.querySelector("p").classList.add(".bg-orange");
});
//jquery 이용한 코딩
$("#changeBtn2").on("click", ()=>{
$("p").text("changed").css("color","red").addClass("bg-orange");
$(".my-class").text("changed").css("color","red").addClass("bg-orange");
$("#one").text("changed").css("color","red").addClass("bg-orange");
});
</script>
</body>
</html>
이제 다시 이클립스 작업으로 넘어간다.


응답하는 send.jsp 페이지의 응답하는 문자열 형식을 json이나 xml을 사용하면
응답하기에 용이하다.



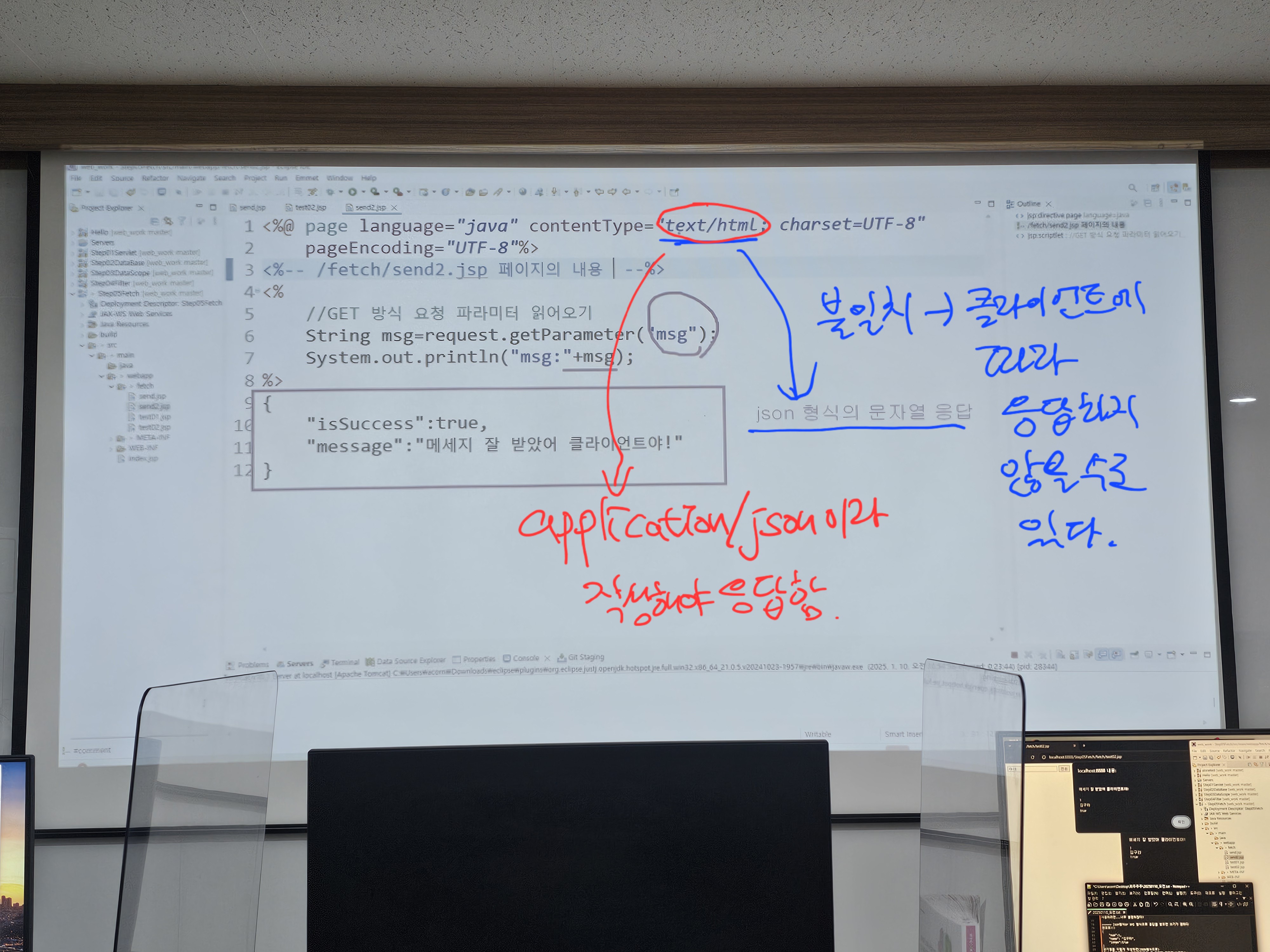
json.parse() 함수를 사용하면 json 문자열인 것을 웹브라우저에서 바로 배열로 바꿔줌
근데 서버에서 res.json()을 사용해서 리턴하면 서버가 알아서 자동으로 parse 해서 전달해줌.
<11:30 3교시>
fetch emmet 저장함
fetch("send.jsp?msg="+msg)
.then(res=>res.text())
.then(data=>{
});
.catch(error=>{
console.log("에러 정보:" +error);
});
<%@ page language="java" contentType="application/json; charset=UTF-8"
pageEncoding="UTF-8"%>
<%-- /fetch/member.jsp 페이지의 내용 --%>
{
"num":1,
"name":"이름1",
"addr":주소1""
}
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>/fetch/test03.jsp</title>
</head>
<body>
<button id="getBtn">회원정보 가져오기</button>
<p>번호 : <strong id="num"></strong></p>
<p>이름 : <strong id="name"></strong></p>
<p>주소 : <strong id="addr"></strong></p>
<script src="https://cdn.jsdelivr.net/npm/jquery@3.7.1/dist/jquery.min.js"></script>
<script>
$("#getBtn").on("click", ()=>{
fetch("member.jsp")
.then(res=>res.json())
.then(data=>{
console.log(data);
// jquery 의 .text() 동작을 이용해서 data object 에 담긴 정보를 innerText 에 출력하기
$("#num").text(data.num);
$("#name").text(data.name);
$("#addr").text(data.addr);
})
.catch(error=>{
console.log("에러정보:"+error);
});
});
</script>
</body>
</html>

<12:30 4교시>
과제 오후에 할 거 각자 해보기
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<button id="getBtn">친구이름 목록 받아오기</button>
<ul id="friendList">
</ul>
</body>
</html>
li 요소를 만들어야하기 때문에 jquery 홈페이지에서 관련 코드들을 찾아봄
https://api.jquery.com/
jQuery API Documentation
jQuery is a fast, small, and feature-rich JavaScript library. It makes things like HTML document traversal and manipulation, event handling, animation, and Ajax much simpler with an easy-to-use API that works across a multitude of browsers. If you're new t
api.jquery.com
https://api.jquery.com/add/#add-elements
.add() | jQuery API Documentation
Description: Create a new jQuery object with elements added to the set of matched elements. Given a jQuery object that represents a set of DOM elements, the .add() method constructs a new jQuery object from the union of those elements and the ones passed i
api.jquery.com
https://api.jquery.com/append/
.append() | jQuery API Documentation
Description: Insert content, specified by the parameter, to the end of each element in the set of matched elements. The .append() method inserts the specified content as the last child of each element in the jQuery collection (To insert it as the first chi
api.jquery.com
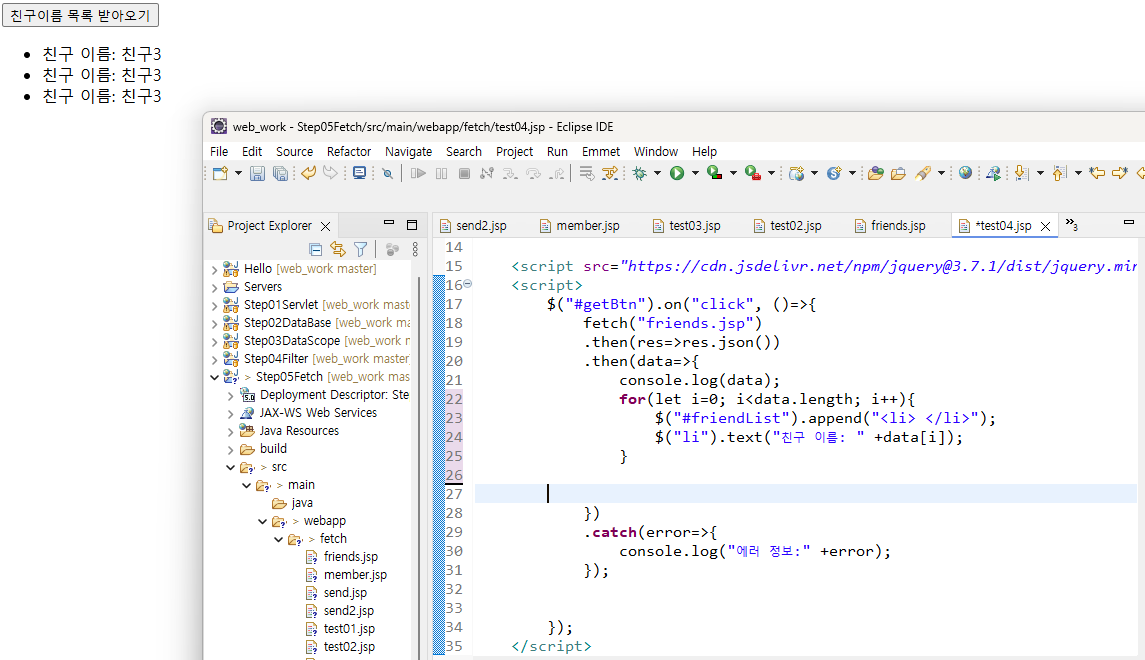
append랑 text 기능 살펴보고 여기까진 혼자 함.
'공부의 기록 > 자바 풀 스택 : 수업내용정리' 카테고리의 다른 글
자바 풀 스택 1/13 오전 기록 036-1 (0) | 2025.01.13 |
---|---|
자바 풀 스택 1/10 오후 기록 035-2 (0) | 2025.01.10 |
자바 풀 스택 1/9 오후 기록 034-2 (0) | 2025.01.09 |
자바 풀 스택 1/9 오전 기록 034-1 (0) | 2025.01.09 |
자바 풀 스택 1/8 오후 기록 033-2 (0) | 2025.01.08 |